rate_functions#
A selection of rate functions, i.e., speed curves for animations. Please find a standard list at https://easings.net/. Here is a picture for the non-standard ones
Example: RateFuncExample ¶
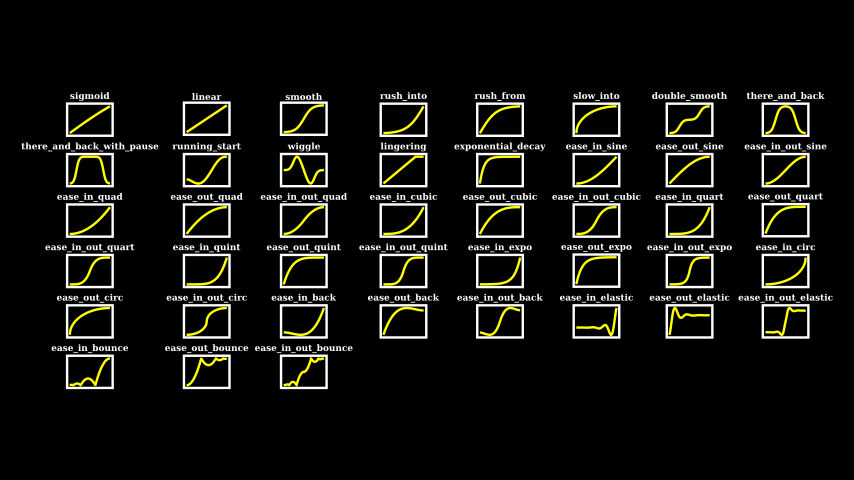
from manim import *
class RateFuncExample(Scene):
def construct(self):
x = VGroup()
for k, v in rate_functions.__dict__.items():
if "function" in str(v):
if (
not k.startswith("__")
and not k.startswith("sqrt")
and not k.startswith("bezier")
):
try:
rate_func = v
plot = (
ParametricFunction(
lambda x: [x, rate_func(x), 0],
t_range=[0, 1, .01],
use_smoothing=False,
color=YELLOW,
)
.stretch_to_fit_width(1.5)
.stretch_to_fit_height(1)
)
plot_bg = SurroundingRectangle(plot).set_color(WHITE)
plot_title = (
Text(rate_func.__name__, weight=BOLD)
.scale(0.5)
.next_to(plot_bg, UP, buff=0.1)
)
x.add(VGroup(plot_bg, plot, plot_title))
except: # because functions `not_quite_there`, `function squish_rate_func` are not working.
pass
x.arrange_in_grid(cols=8)
x.height = config.frame_height
x.width = config.frame_width
x.move_to(ORIGIN).scale(0.95)
self.add(x)
There are primarily 3 kinds of standard easing functions:
Ease In - The animation has a smooth start.
Ease Out - The animation has a smooth end.
Ease In Out - The animation has a smooth start as well as smooth end.
Note
The standard functions are not exported, so to use them you do something like this: rate_func=rate_functions.ease_in_sine On the other hand, the non-standard functions, which are used more commonly, are exported and can be used directly.
Example: RateFunctions1Example ¶
from manim import *
class RateFunctions1Example(Scene):
def construct(self):
line1 = Line(3*LEFT, 3*RIGHT).shift(UP).set_color(RED)
line2 = Line(3*LEFT, 3*RIGHT).set_color(GREEN)
line3 = Line(3*LEFT, 3*RIGHT).shift(DOWN).set_color(BLUE)
dot1 = Dot().move_to(line1.get_left())
dot2 = Dot().move_to(line2.get_left())
dot3 = Dot().move_to(line3.get_left())
label1 = Tex("Ease In").next_to(line1, RIGHT)
label2 = Tex("Ease out").next_to(line2, RIGHT)
label3 = Tex("Ease In Out").next_to(line3, RIGHT)
self.play(
FadeIn(VGroup(line1, line2, line3)),
FadeIn(VGroup(dot1, dot2, dot3)),
Write(VGroup(label1, label2, label3)),
)
self.play(
MoveAlongPath(dot1, line1, rate_func=rate_functions.ease_in_sine),
MoveAlongPath(dot2, line2, rate_func=rate_functions.ease_out_sine),
MoveAlongPath(dot3, line3, rate_func=rate_functions.ease_in_out_sine),
run_time=7
)
self.wait()
Functions
- exponential_decay(t, half_life=0.1)[source]#
- Parameters
t (float) –
half_life (float) –
- Return type
float
- not_quite_there(func=<function smooth>, proportion=0.7)[source]#
- Parameters
func (Callable[[float], float]) –
proportion (float) –
- Return type
Callable[[float], float]
- running_start(t, pull_factor=- 0.5)[source]#
- Parameters
t (float) –
pull_factor (float) –
- Return type
Iterable
- rush_from(t, inflection=10.0)[source]#
- Parameters
t (float) –
inflection (float) –
- Return type
float
- rush_into(t, inflection=10.0)[source]#
- Parameters
t (float) –
inflection (float) –
- Return type
float
- squish_rate_func(func, a=0.4, b=0.6)[source]#
- Parameters
func (Callable[[float], float]) –
a (float) –
b (float) –
- Return type
Callable[[float], float]
- there_and_back(t, inflection=10.0)[source]#
- Parameters
t (float) –
inflection (float) –
- Return type
float