matrix#
Mobjects representing matrices.
Examples
Example: MatrixExamples ¶
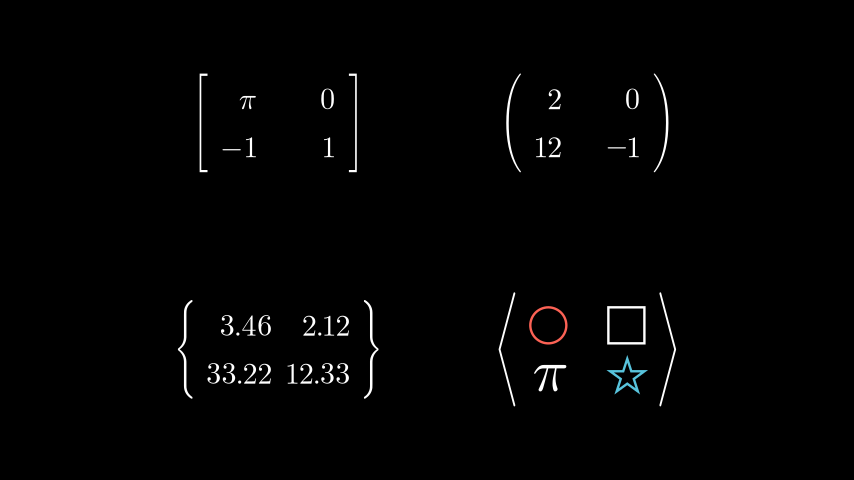
from manim import *
class MatrixExamples(Scene):
def construct(self):
m0 = Matrix([["\\pi", 0], [-1, 1]])
m1 = IntegerMatrix([[1.5, 0.], [12, -1.3]],
left_bracket="(",
right_bracket=")")
m2 = DecimalMatrix(
[[3.456, 2.122], [33.2244, 12.33]],
element_to_mobject_config={"num_decimal_places": 2},
left_bracket="\\{",
right_bracket="\\}")
m3 = MobjectMatrix(
[[Circle().scale(0.3), Square().scale(0.3)],
[MathTex("\\pi").scale(2), Star().scale(0.3)]],
left_bracket="\\langle",
right_bracket="\\rangle")
g = Group(m0, m1, m2, m3).arrange_in_grid(buff=2)
self.add(g)
Classes
A mobject that displays a matrix with decimal entries on the screen. |
|
A mobject that displays a matrix with integer entries on the screen. |
|
A mobject that displays a matrix on the screen. |
|
A mobject that displays a matrix of mobject entries on the screen. |
Functions
- get_det_text(matrix, determinant=None, background_rect=False, initial_scale_factor=2)[source]#
Helper function to create determinant.
- Parameters
matrix (Matrix) – The matrix whose determinant is to be created
determinant (int | str | None) – The value of the determinant of the matrix
background_rect (bool) – The background rectangle
initial_scale_factor (float) – The scale of the text det w.r.t the matrix
- Returns
A VGroup containing the determinant
- Return type
Examples
Example: DeterminantOfAMatrix ¶
from manim import * class DeterminantOfAMatrix(Scene): def construct(self): matrix = Matrix([ [2, 0], [-1, 1] ]) # scaling down the `det` string det = get_det_text(matrix, determinant=3, initial_scale_factor=1) # must add the matrix self.add(matrix) self.add(det)